In this post, we will build a Signup and Login app using Firebase Auth. Firebase helps to develop high-quality apps and their features work independently. Now let’s see how we can build a signup and login app with Firebase database.
Signup and Login auth
App Demo Screens of Signup and Login screen

Now let’s get started to create a new project
Page Contents
1. Steps for creating new project
- Start Android Studio.
- Create a New Project window that opens.
- Select Phone and Tablet.
- Select Empty Activity.
- Click Next.
- Enter the project name/App name.
- Set the company name as desired. In my case, I am using techpassappmaster.sign_upandlog_inwithfirebase
- Then select the project file location is and change it if desired.
- Select language java.
- Select Minimum SDK Android 4.3.
- Click “Finish.
After the project is successfully created then add your project with Firebase.
Note: – Your Android App and firebase project package name should be the same (mine is com.techpassappmaster.sign_upandlog_inwithfirebase).
2. Enabling Firebase Authentication
Go to your firebase project dashboard. Click the» Authentication » Sign-in method and choose Email & Password and enable it.

3. Add Internet Permission
Open AndroidManifest.xml and add INTERNET permission.
<uses-permission android:name="android.permission.INTERNET" />
4. Add Google Services classpath
Open the build.gradle and add Firebase google services dependency.
dependencies { classpath 'com.android.tools.build:gradle:4.0.0' classpath 'com.google.gms:google-services:4.2.0' }
5. Add Firebase auth dependency and Apply Google Services Plugin
Open app/build.gradle and add Firebase authentication dependency. At the very bottom of the file, add apply plugin: ‘com.google.gms.google-services
dependencies { implementation fileTree(dir: 'libs', include: ['*.jar']) implementation 'androidx.appcompat:appcompat:1.0.0' implementation 'androidx.constraintlayout:constraintlayout:1.1.3' testImplementation 'junit:junit:4.12' androidTestImplementation 'androidx.test.ext:junit:1.1.1' androidTestImplementation 'androidx.test.espresso:espresso-core:3.2.0' implementation 'com.google.android.material:material:1.0.0' // firebase auth implementation 'com.google.firebase:firebase-auth:16.0.5' } apply plugin: 'com.google.gms.google-services'
Now we have added all the required dependencies. Let’s start to make the sign-up screen.
6. Create Signup Screen
- Create an activity named SignupActivity and add the following code to the layout file activity_signup.xml.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:orientation="vertical" tools:context=".SignUpActivity"> <ProgressBar android:id="@+id/signUp_progress" android:layout_width="wrap_content" android:layout_height="wrap_content" android:visibility="gone" /> <ImageView android:layout_width="match_parent" android:layout_height="140dp" android:layout_marginBottom="22dp" android:background="@drawable/firebase_logo" /> <TextView android:id="@+id/login_title" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="22dp" android:text="New User Sign Up" android:textAlignment="center" android:textColor="@android:color/black" android:textSize="26sp" android:textStyle="bold" /> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical" android:padding="20dp"> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="wrap_content"> <EditText android:id="@+id/edit_txt_email" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="Email" android:inputType="textWebEmailAddress" /> </com.google.android.material.textfield.TextInputLayout> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="wrap_content" app:passwordToggleEnabled="true"> <EditText android:id="@+id/edit_txt_pass" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="Password" android:inputType="textPassword" /> </com.google.android.material.textfield.TextInputLayout> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="wrap_content" app:passwordToggleEnabled="true"> <EditText android:id="@+id/edit_txt_coPass" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="Co-Password" android:inputType="textPassword" /> </com.google.android.material.textfield.TextInputLayout> <Button android:id="@+id/button_register" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_margin="22dp" android:background="#FFC400" android:text="Sign Up" android:textAllCaps="false" android:textColor="#fff" android:textSize="18sp" /> <TextView android:id="@+id/text_view_login" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:text="Already have an account.\nLogin Here" android:textAlignment="center" android:textColor="@android:color/holo_green_dark" android:textSize="20sp" /> </LinearLayout> </LinearLayout>
- Open SignupActivity.java and add the following code. Firebase provide the createUserWithEmailAndPassword() method for the user authentication.
package com.techpassappmaster.sign_upandlog_inwithfirebase; import android.content.Intent; import android.os.Bundle; import android.text.TextUtils; import android.util.Patterns; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.ProgressBar; import android.widget.TextView; import android.widget.Toast; import androidx.annotation.NonNull; import androidx.appcompat.app.AppCompatActivity; import com.google.android.gms.tasks.OnCompleteListener; import com.google.android.gms.tasks.Task; import com.google.firebase.auth.AuthResult; import com.google.firebase.auth.FirebaseAuth; /** * Created by Techpass Master on 26-Jun-20. * www.techpassmaster.com */ public class SignUpActivity extends AppCompatActivity { // private static final Pattern PASSWORD_PATTERN = Pattern.compile("^" + "(?=.*[0-9])" + "(?=.*[a-z])" + "(?=.*[A-Z])" + "(?=.*[a-zA-Z])" + "(?=.*[@#$%^&+=])" + "(?=\\S+$)" + ".{4,}" + "$"); private EditText txtemail, txtpass, txtcopass; private Button button_register; private TextView text_view_login; private FirebaseAuth mAuth; ProgressBar signUp_progress; String password, co_password, email; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_signup); // get all view id from XML txtemail = findViewById(R.id.edit_txt_email); txtpass = findViewById(R.id.edit_txt_pass); txtcopass = findViewById(R.id.edit_txt_coPass); signUp_progress = findViewById(R.id.signUp_progress); text_view_login = findViewById(R.id.text_view_login); button_register = findViewById(R.id.button_register); // Get Firebase auth instance mAuth = FirebaseAuth.getInstance(); text_view_login.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent intent = new Intent(getApplicationContext(), LoginActivity.class); startActivity(intent); } }); // handle user SignUp button button_register.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (!validateEmail() | !validatePassword()) { return; } if (password.equals(co_password)) { // progressbar VISIBLE signUp_progress.setVisibility(View.VISIBLE); mAuth.createUserWithEmailAndPassword(email, password).addOnCompleteListener(new OnCompleteListener<AuthResult>() { @Override public void onComplete(@NonNull Task<AuthResult> task) { if (task.isSuccessful()) { // progressbar GONE signUp_progress.setVisibility(View.GONE); Toast.makeText(SignUpActivity.this, "Successful Registered", Toast.LENGTH_SHORT).show(); Intent intent = new Intent(SignUpActivity.this, MainActivity.class); startActivity(intent); finish(); } else { // progressbar GONE signUp_progress.setVisibility(View.GONE); Toast.makeText(SignUpActivity.this, "Check Email id or Password", Toast.LENGTH_SHORT).show(); } } }); } else { Toast.makeText(SignUpActivity.this, "Password didn't match", Toast.LENGTH_SHORT).show(); } } }); } private boolean validateEmail() { email = txtemail.getText().toString().trim(); if (TextUtils.isEmpty(email)) { Toast.makeText(SignUpActivity.this, "Enter Your Email", Toast.LENGTH_SHORT).show(); return false; } else if (!Patterns.EMAIL_ADDRESS.matcher(email).matches()) { Toast.makeText(SignUpActivity.this, "Please enter valid Email", Toast.LENGTH_SHORT).show(); return false; } else { return true; } } private boolean validatePassword() { password = txtpass.getText().toString().trim(); co_password = txtcopass.getText().toString().toLowerCase(); if (TextUtils.isEmpty(password)) { Toast.makeText(SignUpActivity.this, "Enter Your Password", Toast.LENGTH_SHORT).show(); return false; } else if (TextUtils.isEmpty(co_password)) { Toast.makeText(SignUpActivity.this, "Enter Your Co-Password", Toast.LENGTH_SHORT).show(); return false; } else if (password.length() <= 6) { Toast.makeText(SignUpActivity.this, "Password is Very Short", Toast.LENGTH_SHORT).show(); return false; } else { return true; } } // if the user already logged in then it will automatically send on dashboard activity. @Override public void onStart() { super.onStart(); if (FirebaseAuth.getInstance().getCurrentUser() != null) { Intent intent = new Intent(SignUpActivity.this, MainActivity.class); startActivity(intent); } } }
- Now, launch Signup screen. and click to run button and test the signup with email and password.
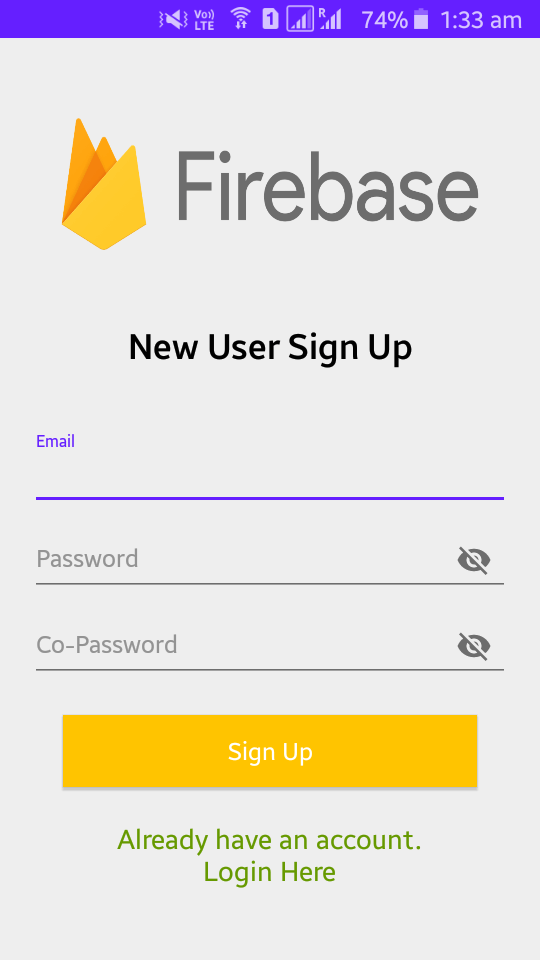
- After the signup screen launched, enter the email id and password, if you successfully login to Firebase you can see the user-created with the email id in the below image which you gave in the app.

7. Create Login Screen with Email & Password
- Create LoginActivity and add the below code to its layout file activity_login.xml.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:orientation="vertical" tools:context=".LoginActivity"> <ProgressBar android:id="@+id/login_progress" android:layout_width="wrap_content" android:layout_height="wrap_content" android:visibility="gone" /> <ImageView android:layout_width="match_parent" android:layout_height="140dp" android:layout_marginBottom="22dp" android:background="@drawable/firebase_logo" /> <TextView android:id="@+id/login_title" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="22dp" android:text="Login Account" android:textAlignment="center" android:textColor="@android:color/black" android:textSize="26sp" android:textStyle="bold" /> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="vertical" android:padding="20dp"> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="wrap_content"> <EditText android:id="@+id/edit_txt_login_email" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="Email" android:inputType="textWebEmailAddress" /> </com.google.android.material.textfield.TextInputLayout> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="wrap_content" app:passwordToggleEnabled="true"> <EditText android:id="@+id/edit_txt_login_pass" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="Password" android:inputType="textPassword" /> </com.google.android.material.textfield.TextInputLayout> <TextView android:id="@+id/text_view_forget_password" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="right" android:paddingTop="8dp" android:text="Forgot Password ?" android:textColor="#651FFF" android:textSize="16sp" /> <Button android:id="@+id/button_login" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_margin="22dp" android:background="#FFC400" android:text="Login" android:textAllCaps="false" android:textColor="#fff" android:textSize="18sp" /> <TextView android:id="@+id/text_view_signup" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:text="Don't have an account.\nSign Up Here" android:textAlignment="center" android:textColor="@android:color/holo_green_dark" android:textSize="20sp" /> </LinearLayout> </LinearLayout>
- Open LoginActivity.java and add the below code. Firebase gives signInWithEmailAndPassword()method to sign in the user.
package com.techpassappmaster.sign_upandlog_inwithfirebase; import android.content.Intent; import android.os.Bundle; import android.text.TextUtils; import android.util.Patterns; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.ProgressBar; import android.widget.TextView; import android.widget.Toast; import androidx.annotation.NonNull; import androidx.appcompat.app.AppCompatActivity; import com.google.android.gms.tasks.OnCompleteListener; import com.google.android.gms.tasks.Task; import com.google.firebase.auth.AuthResult; import com.google.firebase.auth.FirebaseAuth; /** * Created by Techpass Master on 26-Jun-20. * www.techpassmaster.com */ public class LoginActivity extends AppCompatActivity { private EditText txtemail, txtpassoword; private Button login_btn; private TextView text_view_signup, forgot_password; ProgressBar login_progress; FirebaseAuth mAuth; String loginemail, loginpassword; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_login); // get all view id from XML txtemail = findViewById(R.id.edit_txt_login_email); txtpassoword = findViewById(R.id.edit_txt_login_pass); forgot_password = findViewById(R.id.text_view_forget_password); login_progress = findViewById(R.id.login_progress); text_view_signup = findViewById(R.id.text_view_signup); login_btn = findViewById(R.id.button_login); // Get Firebase auth instance mAuth = FirebaseAuth.getInstance(); // handle login button login_btn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (!validateEmail() | !validatePassword()) { return; } // progressbar VISIBLE login_progress.setVisibility(View.VISIBLE); mAuth.signInWithEmailAndPassword(loginemail, loginpassword). addOnCompleteListener(new OnCompleteListener<AuthResult>() { @Override public void onComplete(@NonNull Task<AuthResult> task) { // progressbar GONE login_progress.setVisibility(View.GONE); if (task.isSuccessful()) { Toast.makeText(LoginActivity.this, "Login Successful", Toast.LENGTH_SHORT).show(); Intent intent = new Intent(LoginActivity.this, MainActivity.class); startActivity(intent); finish(); } else { // progressbar GONE login_progress.setVisibility(View.GONE); Toast.makeText(LoginActivity.this, "Login Failed", Toast.LENGTH_SHORT).show(); } } }); } }); // handle forgot button forgot_password.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent intent = new Intent(getApplicationContext(), ForgotPasswordActivity.class); startActivity(intent); } }); // handle signUp textview text_view_signup.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent intent = new Intent(getApplicationContext(), SignUpActivity.class); startActivity(intent); } }); } private boolean validateEmail() { loginemail = txtemail.getText().toString().trim(); if (TextUtils.isEmpty(loginemail)) { Toast.makeText(LoginActivity.this, "Enter Your Email", Toast.LENGTH_SHORT).show(); return false; } else if (!Patterns.EMAIL_ADDRESS.matcher(loginemail).matches()) { Toast.makeText(LoginActivity.this, "Please enter valid Email", Toast.LENGTH_SHORT).show(); return false; } else { return true; } } private boolean validatePassword() { loginpassword = txtpassoword.getText().toString().trim(); if (TextUtils.isEmpty(loginpassword)) { Toast.makeText(LoginActivity.this, "Enter Your Password", Toast.LENGTH_SHORT).show(); return false; } else { return true; } } }
- New, launch LoginActivity.java. Run the project and login with the credentials that you want to use while signing up.
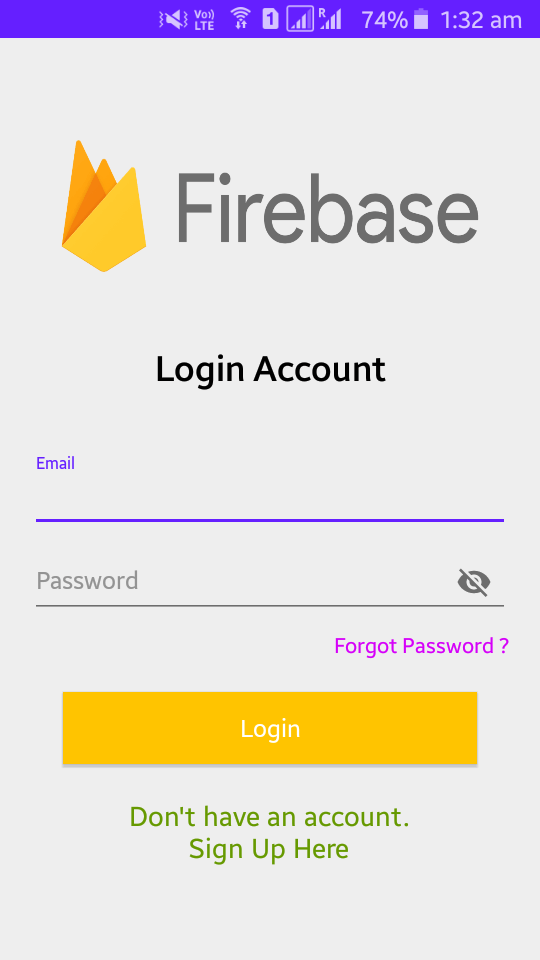
8. Create Forgot Password Screen
Send Reset Password Link on the Email:
Firebase provides the feature to reset the password. When you forget your auth password then you have a need to your auth email because Firebase sends a reset password URL link on your email account. So let’s see the code of it.
- Create another activity named ForgotPasswordActivity.java and add the below code its layout file activity_forgot_password.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:orientation="vertical" tools:context=".ForgotPasswordActivity"> <ProgressBar android:id="@+id/resetPassword_progress" android:layout_width="wrap_content" android:visibility="gone" android:layout_height="wrap_content"/> <ImageView android:layout_width="match_parent" android:layout_height="140dp" android:layout_marginBottom="22dp" android:background="@drawable/firebase_logo" /> <TextView android:id="@+id/login_title" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Reset Password ?" android:textAlignment="center" android:layout_marginBottom="22dp" android:textColor="@android:color/black" android:textSize="26sp" android:textStyle="bold" /> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Enter your email to received reset link" android:textAlignment="center" android:layout_marginBottom="22dp" android:textColor="@android:color/black" android:textSize="20sp" /> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:padding="20dp" android:orientation="vertical"> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="wrap_content"> <EditText android:id="@+id/edit_txt_resetEmail" android:layout_width="match_parent" android:layout_height="wrap_content" android:hint="Email" android:inputType="textWebEmailAddress" /> </com.google.android.material.textfield.TextInputLayout> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="wrap_content" app:passwordToggleEnabled="true"/> <com.google.android.material.textfield.TextInputLayout android:layout_width="match_parent" android:layout_height="wrap_content" app:passwordToggleEnabled="true"/> <Button android:id="@+id/button_resetPassword" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_margin="22dp" android:background="#FFC400" android:text="Send Me Link" android:textAllCaps="false" android:textColor="#fff" android:textSize="20sp" /> </LinearLayout> </LinearLayout>
- Open ForgotPasswordActivity.java. You can use the sendPasswordResetEmail() method to send the password reset URL link on your email, follow the below code.
package com.techpassappmaster.sign_upandlog_inwithfirebase; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.ProgressBar; import android.widget.Toast; import androidx.annotation.NonNull; import androidx.appcompat.app.AppCompatActivity; import com.google.android.gms.tasks.OnCompleteListener; import com.google.android.gms.tasks.Task; import com.google.firebase.auth.FirebaseAuth; /** * Created by Techpass Master on 26-Jun-20. * www.techpassmaster.com */ public class ForgotPasswordActivity extends AppCompatActivity { private EditText edit_txt_resetEmail; private Button button_resetPassword; private ProgressBar resetPassword_progress; FirebaseAuth firebaseAuth; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_forgot_password); // get all view id from XML edit_txt_resetEmail = findViewById(R.id.edit_txt_resetEmail); button_resetPassword = findViewById(R.id.button_resetPassword); resetPassword_progress = findViewById(R.id.resetPassword_progress); // Get Firebase auth instance firebaseAuth = FirebaseAuth.getInstance(); button_resetPassword.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { resetPassword_progress.setVisibility(View.VISIBLE); firebaseAuth.sendPasswordResetEmail(edit_txt_resetEmail.getText().toString()) .addOnCompleteListener(new OnCompleteListener<Void>() { @Override public void onComplete(@NonNull Task<Void> task) { resetPassword_progress.setVisibility(View.GONE); if (task.isSuccessful()) { Toast.makeText(ForgotPasswordActivity.this, "Password reset link sent to your Email", Toast.LENGTH_SHORT).show(); Intent intent = new Intent(ForgotPasswordActivity.this, LoginActivity.class); startActivity(intent); finish(); } else { Toast.makeText(ForgotPasswordActivity.this, "Something error", Toast.LENGTH_SHORT).show(); } } }); } }); } }
- Now, launch ForgotPasswordActivity. Run and test the reset password.

This is your reset password URL. Click to change the new password.

9. Create Logout Screen
- After the successful login, the user will be switch to the dashboard or main activity, for that create a new activity named MainActivity.
- Add the below code its layout file activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:gravity="center" android:orientation="vertical" android:padding="16sp" tools:context=".MainActivity"> <TextView android:id="@+id/txt_userEmail" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="User Email" android:textAlignment="center" android:textColor="#02C164" android:textSize="26sp" android:textStyle="bold" /> <Button android:id="@+id/btn_userlogout" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="100dp" android:background="#651FFF" android:text="Logout" android:textColor="#ffff" android:textSize="18sp" android:textStyle="bold" /> </LinearLayout>
- Open MainActivity.java. You can use the signOut() method for the logout credentials user account and add the following code to MainActivity.java.
package com.techpassappmaster.sign_upandlog_inwithfirebase; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.ImageView; import android.widget.TextView; import android.widget.Toast; import androidx.appcompat.app.AppCompatActivity; import com.google.firebase.auth.FirebaseAuth; import com.google.firebase.auth.FirebaseUser; /** * Created by Techpass Master on 26-Jun-20. * www.techpassmaster.com */ public class MainActivity extends AppCompatActivity { private TextView txt_userEmail; private Button btn_userlogout; FirebaseUser firebaseUser; FirebaseAuth mAuth; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // get all view id from XML txt_userEmail = findViewById(R.id.txt_userEmail); btn_userlogout = findViewById(R.id.btn_userlogout); // Get Firebase auth instance mAuth = FirebaseAuth.getInstance(); firebaseUser = mAuth.getCurrentUser(); txt_userEmail.setText(firebaseUser.getEmail()); // handle Logout button btn_userlogout.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { mAuth.signOut(); Toast.makeText(MainActivity.this, "Logout Successful ", Toast.LENGTH_SHORT).show(); Intent intent = new Intent(MainActivity.this, LoginActivity.class); startActivity(intent); } }); } @Override public void onBackPressed() { super.onBackPressed(); finishAffinity(); } }
10. Click Run button and test the Logout.

Read More:
- Android Working With Firebase – Signup And Login Auth.
- Getting Started With Firebase On Android.
- Android Interview Questions For Fresher 2021.
- Top 5 Things To Avoid While Developing Android Apps.
Congrats! You’ve now completed your signup and login app with Firebase. Check the demo app link given below.
I’m extremely impressed with your writing skills as well as with
the layout on your blog. Is this a paid theme or
did you modify it yourself? Either way keep up the excellent quality writing, it is rare
to see a nice blog like this one these days.
Modify it himself and it’s not paid theme. Thank you
Hi there, I enjoy reading through your article post. I wanted to write a little comment to support you.
Thank you
Hello, There’s no doubt that your web site might be having web browser compatibility issues. Whenever I take a look at your blog in Safari, it looks fine but when opening in Internet Explorer, it’s got some overlapping issues. I just wanted to provide you with a quick heads up! Apart from that, excellent blog!
we will try to fix it ASAP
Thanks to my father who told me about this weblog, this weblog is actually remarkable.
Appreciating the time and energy you put into your website and detailed information you provide. It’s good to come across a blog every once in a while that isn’t the same out of date rehashed information. Fantastic read! I’ve saved your site and I’m including your RSS feeds to my Google account.
What’s up, everything is going sound here and ofcourse every one is sharing information, that’s really excellent, keep up writing.
I am regular visitor, how are you everybody? This article posted at this web page is actually good.
Very good article! We will be linking to this great post on our site. Keep up the good writing.
Hi I am so glad I found your web site, I really found you by error, while I was researching on Yahoo for something else, Anyways I am here now and would just like to say cheers for a tremendous post and a all round interesting blog (I also love the theme/design), I don’t have time to browse it all at the moment but I have bookmarked it and also included your RSS feeds, so when I have time I will be back to read a great deal more, Please do keep up the superb work.
Hi to all, the contents present at this site are genuinely amazing for people knowledge, well, keep up the nice work fellows.
It’s the best time to make some plans for the future and it’s time to be happy. I have read this post and if I could I desire to suggest you some interesting things or advice. Maybe you could write next articles referring to this article. I desire to read more things about it!
Hello There. I discovered your blog the usage of msn. That is an extremely well written article. I will make sure to bookmark it and return to learn more of your helpful information. Thanks for the post. I’ll certainly comeback.
Everything is very open with a clear explanation of the issues.
It was definitely informative.
Your site is very useful.
Thanks for sharing!
Thanks, Sabine 🙂